Chapter 7
Working with Objects
JavaScript
is designed on a simple object-based paradigm. An object is a construct
with properties that are JavaScript variables or other objects. An object
also has functions associated with it that are known as the object's
methods. In addition to objects that are predefined in the
Navigator client and the server, you can define your own objects.
This
chapter describes how to use objects, properties, functions, and
methods, and how to create your own objects.
This
chapter contains the following sections:
Objects and Properties
A JavaScript
object has properties associated with it. You access the properties of an
object with a simple notation:
objectName.propertyName
Both the
object name and property name are case sensitive. You define a property
by assigning it a value. For example, suppose there is an object named
myCar (for
now, just assume the object already exists). You can give it properties
named make,
model, and
year as
follows:
myCar.make = "Ford";
myCar.model = "Mustang";
myCar.year = 1969;
An array
is an ordered set of values associated with a single variable name.
Properties and arrays in JavaScript are intimately related; in fact,
they are different interfaces to the same data structure. So, for
example, you could access the properties of the myCar object as follows:
myCar["make"] = "Ford"
myCar["model"] = "Mustang"
myCar["year"] = 1967
This type
of array is known as an associative array, because each index
element is also associated with a string value. To illustrate how this
works, the following function displays the properties of the object
when you pass the object and the object's name as arguments to the
function:
function show_props(obj, obj_name) {
var result = "";
for (var i in obj)
result += obj_name + "." + i + " =
" + obj[i] + "\n";
return result
}
So, the
function call show_props(myCar,
"myCar") would return the following:
myCar.make = Ford
myCar.model = Mustang
myCar.year = 1967
Creating
New Objects
JavaScript
has a number of predefined objects. In addition, you can create your own
objects. In JavaScript 1.2 and later, you can create an object using
an object initializer. Alternatively, you can first create a constructor
function and then instantiate an object using that function and the
new operator.
Using Object Initializers
In
addition to creating objects using a constructor function, you can
create objects using an object initializer. Using object initializers
is sometimes referred to as creating objects with literal notation.
"Object initializer" is consistent with the terminology used by C++.
The syntax
for an object using an object initializer is:
objectName = {property1:value1,
property2:value2,..., propertyN:valueN}
where
objectName is
the name of the new object, each property
I
is an identifier (either a name, a number, or a string literal),
and each value I is an
expression whose value is assigned to the property
I.
The objectName and
assignment is optional. If you do not need to refer to this
object elsewhere, you do not need to assign it to a variable.
If an
object is created with an object initializer in a top-level script,
JavaScript interprets the object each time it evaluates the expression
containing the object literal. In addition, an initializer used in a
function is created each time the function is called.
The
following statement creates an object and assigns it to the variable
x if and only
if the expression cond is true.
if
(cond) x = {hi:"there"}
The
following example creates myHonda with three
properties. Note that the engine property is also
an object with its own properties.
myHonda =
{color:"red",wheels:4,engine:{cylinders:4,size:2.2}}
You can
also use object initializers to create arrays. See "Array Literals" on page 28.
JavaScript 1.1 and earlier.
You cannot
use object initializers. You can create objects only using their
constructor functions or using a function supplied by some other object
for that purpose. See Using a Constructor
Function.
Using a Constructor Function
Alternatively, you can create an object with these
two steps:
-
-
Define the object type by writing a constructor
function.
-
Create an instance of the object with
new.
To define an
object type, create a function for the object type that specifies its
name, properties, and methods. For example, suppose you want to create an
object type for cars. You want this type of object to be called
car, and you
want it to have properties for make, model, year, and color. To do this,
you would write the following function:
function car(make, model, year) {
this.make = make;
this.model = model;
this.year = year;
}
Notice the
use of this to
assign values to the object's properties based on the values passed to
the function.
Now you
can create an object called mycar as follows:
mycar = new car("Eagle", "Talon TSi", 1993);
This
statement creates mycar and assigns it the
specified values for its properties. Then the value of
mycar.make
is the string "Eagle", mycar.year is the
integer 1993, and so on.
You can
create any number of car objects by calls to
new. For
example,
kenscar = new car("Nissan", "300ZX", 1992);
vpgscar = new car("Mazda", "Miata", 1990);
An object
can have a property that is itself another object. For example, suppose
you define an object called person as follows:
function person(name, age, sex) {
this.name = name
this.age = age
this.sex = sex
}
and then
instantiate two new person objects as
follows:
rand = new person("Rand McKinnon", 33, "M");
ken = new person("Ken Jones", 39, "M");
Then you
can rewrite the definition of car to include an
owner property
that takes a person object, as
follows:
function car(make, model, year, owner) {
this.make = make;
this.model = model;
this.year = year;
this.owner = owner
}
To
instantiate the new objects, you then use the following:
car1 = new car("Eagle", "Talon TSi", 1993,
rand);
car2 = new car("Nissan", "300ZX", 1992, ken);
Notice
that instead of passing a literal string or integer value when creating
the new objects, the above statements pass the objects
rand and
ken as
the arguments for the owners. Then if you want to find out the
name of the owner of car2, you can access the following property:
car2.owner.name
Note that
you can always add a property to a previously defined object. For
example, the statement
car1.color = "black"
adds a
property color
to car1, and assigns it a value of "black." However, this does not
affect any other objects. To add the new property to all objects of the
same type, you have to add the property to the definition of the
car object
type.
Indexing Object Properties
In
JavaScript 1.0, you can refer to an object's properties by their
property name or by their ordinal index. In JavaScript 1.1 or later,
however, if you initially define a property by its name, you must
always refer to it by its name, and if you initially define a property
by an index, you must always refer to it by its index.
This
applies when you create an object and its properties with a constructor
function, as in the above example of the Car object type, and when
you define individual properties explicitly (for example,
myCar.color =
"red"). So if you define object properties initially with
an index, such as myCar[5] = "25
mpg", you can subsequently refer to the property as
myCar[5].
The
exception to this rule is objects reflected from HTML, such as the
forms array.
You can always refer to objects in these arrays by either their ordinal
number (based on where they appear in the document) or their name (if
defined). For example, if the second <FORM> tag in a
document has a NAME attribute of
"myForm", you can refer to the form as document.forms[1] or
document.forms["myForm"]
or document.myForm.
Defining
Properties for an Object Type
You can
add a property to a previously defined object type by using the
prototype
property. This defines a property that is shared by all objects of the
specified type, rather than by just one instance of the object. The
following code adds a color property to all
objects of type car, and then assigns a
value to the color property of the
object car1.
Car.prototype.color=null;
car1.color="black";
See the
prototype
property of the Function object in the
Core
JavaScript Reference for more information.
Defining Methods
A
method is a function associated with an object. You define a
method the same way you define a standard function. Then you use the
following syntax to associate the function with an existing object:
object.methodname = function_name
where
object is an
existing object, methodname is the name
you are assigning to the method, and function_name is the name
of the function.
You can
then call the method in the context of the object as follows:
object.methodname(params);
You can
define methods for an object type by including a method definition in
the object constructor function. For example, you could define a
function that would format and display the properties of the
previously-defined car objects; for example,
function displayCar() {
var result = "A Beautiful " + this.year + " " +
this.make
+ " " + this.model;
pretty_print(result);
}
where
pretty_print
is function to display a horizontal rule and a string. Notice the use
of this to
refer to the object to which the method belongs.
You can
make this function a method of car by adding the
statement
this.displayCar = displayCar;
to the
object definition. So, the full definition of car would now look like
function car(make, model, year, owner) {
this.make = make;
this.model = model;
this.year = year;
this.owner = owner;
this.displayCar = displayCar;
}
Then you
can call the displayCar method for
each of the objects as follows:
car1.displayCar()
car2.displayCar()
This
produces the output shown in the following figure.
Figure 7.1 Displaying method
output
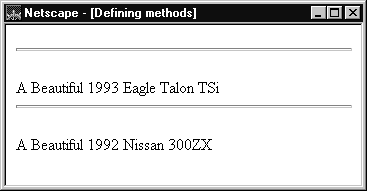
Using this
for Object References
JavaScript has a special keyword,
this,
that you can use within a method to refer to the current
object. For example, suppose you have a function called
validate that
validates an object's value property,
given the object and the high and low values:
function validate(obj, lowval, hival) {
if ((obj.value < lowval) || (obj.value >
hival))
alert("Invalid Value!")
}
Then,
you could call validate in each form
element's onChange event handler,
using this
to pass it the form element, as in the following example:
<INPUT TYPE="text" NAME="age" SIZE=3
onChange="validate(this, 18, 99)">
In
general, this refers to the
calling object in a method.
When
combined with the form property,
this can
refer to the current object's parent form. In the following example,
the form myForm contains a
Text object
and a button. When the user clicks the button, the value of the
Text object
is set to the form's name. The button's onClick event handler
uses this.form to refer to
the parent form, myForm.
<FORM NAME="myForm">
Form name:<INPUT TYPE="text" NAME="text1" VALUE="Beluga">
<P>
<INPUT NAME="button1" TYPE="button" VALUE="Show Form Name"
onClick="this.form.text1.value=this.form.name">
</FORM>
Defining Getters and Setters
A getter
is a method that gets the value of a specific property. A setter is a
method that sets the value of a specific property. You can define
getters and setters on any predefined core object or user-defined
object that supports the addition of new properties. The syntax for
defining getters and setters uses the object literal syntax.
The
following JS shell session illustrates how getters and setters could
work for a user-defined object o. The JS shell is an
application that allows developers to test JavaScript code in batch
mode or interactively.
The
o object's
properties are:
This
JavaScript shell session illustrates how getters and setters can extend
the Date
prototype to add a year property to all instances of the predefined
Date class. It
uses the Date
class's existing getFullYear and
setFullYear
methods to support the year property's getter
and setter.
These
statements define a getter and setter for the year property.:
js> var d = Date.prototype;
js> d.year getter= function() { return this.getFullYear(); };
js> d.year setter= function(y) { return
this.setFullYear(y); };
These
statements use the getter and setter in a Date object:
js> var now = new Date;
js> print(now.year);
2000
js> now.year=2001;
987617605170
js> print(now);
Wed Apr 18 11:13:25 GMT-0700 (Pacific Daylight Time) 2001
Deleting
Properties
You can
remove a property by using the delete operator. The
following code shows how to remove a property.
//Creates a new
property, myobj, with two properties, a and b.
myobj = new Object;
myobj.a=5;
myobj.b=12;
//Removes the a
property, leaving myobj with only the b property.
delete myobj.a;
You can
also use delete to delete a global variable if the var keyword
was not used to declare the variable:
g = 17;
delete g;
See
"delete" on page 46 for more
information.
Predefined Core Objects
This
section describes the predefined objects in core JavaScript:
Array,
Boolean,
Date,
Function,
Math,
Number,
RegExp,
and String.
Array Object
JavaScript does not have an explicit array data
type. However, you can use the predefined Array object and its
methods to work with arrays in your applications. The
Array
object has methods for manipulating arrays in various ways,
such as joining, reversing, and sorting them. It has a property
for determining the array length and other properties for use
with regular expressions.
An
array is an ordered set of values that you refer to with a
name and an index. For example, you could have an array called
emp that
contains employees' names indexed by their employee number. So
emp[1] would
be employee number one, emp[2] employee number
two, and so on.
Creating an
Array
To
create an Array object:
1. arrayObjectName = new Array(element0,
element1, ..., elementN)
2. arrayObjectName = new Array(arrayLength)
arrayObjectName is
either the name of a new object or a property of an existing object.
When using Array properties and
methods, arrayObjectName is
either the name of an existing Array object or a
property of an existing object.
element0, element1,
..., elementN is a list of
values for the array's elements. When this form is specified, the
array is initialized with the specified values as its elements, and
the array's length property is set
to the number of arguments.
arrayLength
is the initial length of the array. The following code creates an
array of five elements:
billingMethod = new Array(5)
Array
literals are also Array objects; for
example, the following literal is an Array object. See
"Array Literals" on page 28 for
details on array literals.
coffees = ["French Roast", "Columbian", "Kona"]
Populating an
Array
You can
populate an array by assigning values to its elements. For example,
emp[1] = "Casey Jones"
emp[2] = "Phil Lesh"
emp[3] = "August West"
You can
also populate an array when you create it:
myArray = new Array("Hello", myVar, 3.14159)
Referring to Array Elements
You
refer to an array's elements by using the element's ordinal number.
For example, suppose you define the following array:
myArray = new Array("Wind","Rain","Fire")
You then
refer to the first element of the array as myArray[0] and the
second element of the array as myArray[1].
The
index of the elements begins with zero (0), but the length of array
(for example, myArray.length)
reflects the number of elements in the array.
Array Methods
The
Array object
has the following methods:
-
-
concat joins two
arrays and returns a new array.
-
join joins all
elements of an array into a string.
-
pop removes the last
element from an array and returns that element.
-
push adds one or more
elements to the end of an array and returns that last element
added.
-
reverse transposes
the elements of an array: the first array element becomes the last
and the last becomes the first.
-
shift removes the
first element from an array and returns that element
-
slice extracts a
section of an array and returns a new array.
-
splice adds and/or
removes elements from an array.
-
sort sorts the
elements of an array.
-
unshift adds one or
more elements to the front of an array and returns the new length
of the array.
For
example, suppose you define the following array:
myArray = new Array("Wind","Rain","Fire")
myArray.join() returns
"Wind,Rain,Fire"; myArray.reverse
transposes the array so that myArray[0] is "Fire",
myArray[1]
is "Rain", and myArray[2] is "Wind".
myArray.sort
sorts the array so that myArray[0] is "Fire",
myArray[1]
is "Rain", and myArray[2] is "Wind".
Two-Dimensional
Arrays
The
following code creates a two-dimensional array.
a
= new Array(4)
for (i=0; i < 4; i++) {
a[i] = new Array(4)
for (j=0; j < 4; j++) {
a[i][j] = "["+i+","+j+"]"
}
}
This
example creates an array with the following rows:
Row 0:[0,0][0,1][0,2][0,3]
Row 1:[1,0][1,1][1,2][1,3]
Row 2:[2,0][2,1][2,2][2,3]
Row 3:[3,0][3,1][3,2][3,3]
Arrays and Regular
Expressions
When an
array is the result of a match between a regular expression and a
string, the array returns properties and elements that provide
information about the match. An array is the return value of
RegExp.exec,
String.match, and
String.split. For
information on using arrays with regular expressions, see Chapter 4, "Regular Expressions."
Boolean Object
The
Boolean
object is a wrapper around the primitive Boolean data type. Use the
following syntax to create a Boolean object:
booleanObjectName = new Boolean(value)
Do not
confuse the primitive Boolean values true and
false
with the true and false values of the Boolean object.
Any object whose value is not undefined ,
null,
0, NaN, or the empty
string , including a Boolean object
whose value is false, evaluates to true when passed to a
conditional statement. See "if...else Statement" on
page 68 for more information.
Date Object
JavaScript does not have a date data type.
However, you can use the Date object and its
methods to work with dates and times in your applications. The
Date object
has a large number of methods for setting, getting, and manipulating
dates. It does not have any properties.
JavaScript handles dates similarly to Java. The
two languages have many of the same date methods, and both languages
store dates as the number of milliseconds since January 1, 1970,
00:00:00.
The
Date object
range is -100,000,000 days to 100,000,000 days relative to 01
January, 1970 UTC.
To
create a Date object:
dateObjectName = new Date([parameters])
where
dateObjectName is the
name of the Date object being
created; it can be a new object or a property of an existing object.
The
parameters
in the preceding syntax can be any of the following:
-
-
Nothing: creates today's date and time. For
example, today =
new Date().
-
A string representing a date in the following
form: "Month day, year hours:minutes:seconds." For example,
Xmas95 = new
Date("December 25, 1995 13:30:00"). If you omit hours,
minutes, or seconds, the value will be set to zero.
-
A set of integer values for year, month, and
day. For example, Xmas95 = new
Date(1995,11,25). A set of values for year, month, day,
hour, minute, and seconds. For example, Xmas95 = new
Date(1995,11,25,9,30,0).
JavaScript 1.2 and earlier.
The
Date object
behaves as follows:
-
-
Dates prior to 1970 are not allowed.
-
JavaScript depends on platform-specific date
facilities and behavior; the behavior of the Date object varies
from platform to platform.
Methods
of the Date Object
The
Date object
methods for handling dates and times fall into these broad
categories:
-
-
"set" methods, for setting date and time
values in Date objects.
-
"get" methods, for getting date and time
values from Date objects.
-
"to" methods, for returning string values
from Date
objects.
-
parse and UTC methods, for parsing
Date
strings.
With the
"get" and "set" methods you can get and set seconds, minutes, hours,
day of the month, day of the week, months, and years separately. There
is a getDay
method that returns the day of the week, but no corresponding
setDay method,
because the day of the week is set automatically. These methods use
integers to represent these values as follows:
-
-
Seconds and minutes: 0 to 59
-
Hours: 0 to 23
-
Day: 0 (Sunday) to 6 (Saturday)
-
Date: 1 to 31 (day of the month)
-
Months: 0 (January) to 11 (December)
-
Year: years since 1900
For
example, suppose you define the following date:
Xmas95 = new Date("December 25, 1995")
Then
Xmas95.getMonth()
returns 11, and Xmas95.getFullYear()
returns 1995.
The
getTime and
setTime
methods are useful for comparing dates. The getTime method returns
the number of milliseconds since January 1, 1970, 00:00:00 for a
Date object.
For
example, the following code displays the number of days left in the
current year:
today = new Date()
endYear = new Date(1995,11,31,23,59,59,999) // Set day and month
endYear.setFullYear(today.getFullYear()) // Set year to this
year
msPerDay = 24 * 60 * 60 * 1000 // Number of milliseconds per day
daysLeft = (endYear.getTime() - today.getTime()) / msPerDay
daysLeft = Math.round(daysLeft) //returns days left in the year
This
example creates a Date object named
today that
contains today's date. It then creates a Date object named
endYear and
sets the year to the current year. Then, using the number of
milliseconds per day, it computes the number of days between today
and endYear,
using getTime and rounding to
a whole number of days.
The
parse method
is useful for assigning values from date strings to existing
Date
objects. For example, the following code uses parse and
setTime
to assign a date value to the IPOdate object:
IPOdate = new Date()
IPOdate.setTime(Date.parse("Aug 9, 1995"))
Using the Date
Object: an Example
In the
following example, the function JSClock() returns the
time in the format of a digital clock.
function JSClock() {
var time = new Date()
var hour = time.getHours()
var minute = time.getMinutes()
var second = time.getSeconds()
var temp = "" + ((hour > 12) ? hour - 12 :
hour)
if (hour == 0)
temp = "12";
temp += ((minute < 10) ? ":0" : ":") +
minute
temp += ((second < 10) ? ":0" : ":") +
second
temp += (hour >= 12) ? " P.M." : " A.M."
return temp
}
The
JSClock
function first creates a new Date object called
time; since
no arguments are given, time is created with the current date and
time. Then calls to the getHours,
getMinutes,
and getSeconds
methods assign the value of the current hour, minute and
seconds to hour, minute, and
second.
The next
four statements build a string value based on the time. The first
statement creates a variable temp, assigning it a
value using a conditional expression; if hour is greater than
12, (hour -
12), otherwise simply hour, unless hour is 0,
in which case it becomes 12.
The next
statement appends a minute value to
temp. If the
value of minute is less than 10,
the conditional expression adds a string with a preceding zero;
otherwise it adds a string with a demarcating colon. Then a statement
appends a seconds value to temp in the same way.
Finally,
a conditional expression appends "PM" to temp if
hour
is 12 or greater; otherwise, it appends "AM" to temp.
Function Object
The
predefined Function object
specifies a string of JavaScript code to be compiled as a function.
To
create a Function object:
functionObjectName = new Function ([arg1, arg2,
... argn], functionBody)
functionObjectName is
the name of a variable or a property of an existing object. It can
also be an object followed by a lowercase event handler name, such as
window.onerror.
arg1, arg2, ...
argn are arguments to be used by the function as formal
argument names. Each must be a string that corresponds to a valid
JavaScript identifier; for example "x" or "theForm".
functionBody
is a string specifying the JavaScript code to be compiled as the
function body.
Function
objects are evaluated each time they are used. This is less efficient
than declaring a function and calling it within your code, because
declared functions are compiled.
In
addition to defining functions as described here, you can also use
the function
statement and the function expression. See the Core
JavaScript Reference for more information.
The
following code assigns a function to the variable setBGColor. This
function sets the current document's background color.
var setBGColor = new
Function("document.bgColor='antiquewhite'")
To call
the Function
object, you can specify the variable name as if it were a function.
The following code executes the function specified by the
setBGColor
variable:
var colorChoice="antiquewhite"
if (colorChoice=="antiquewhite") {setBGColor()}
You can
assign the function to an event handler in either of the following
ways:
1.
document.form1.colorButton.onclick=setBGColor
2. <INPUT NAME="colorButton" TYPE="button"
VALUE="Change background
color"
onClick="setBGColor()">
Creating
the variable setBGColor shown above
is similar to declaring the following function:
function setBGColor() {
document.bgColor='antiquewhite'
}
Assigning a function to a variable is similar to
declaring a function, but there are differences:
-
-
When you assign a function to a variable
using var
setBGColor = new Function("..."), setBGColor is a
variable for which the current value is a reference to the function
created with new
Function().
-
When you create a function using
function
setBGColor() {...}, setBGColor is
not a variable, it is the name of a function.
You can
nest a function within a function. The nested (inner) function is
private to its containing (outer) function:
-
-
The inner function can be accessed only from
statements in the outer function.
-
The inner function can use the arguments and
variables of the outer function. The outer function cannot use the
arguments and variables of the inner function.
Math Object
The
predefined Math object has
properties and methods for mathematical constants and functions. For
example, the Math object's
PI property
has the value of pi (3.141...), which you would use in an application
as
Math.PI
Similarly, standard mathematical functions are
methods of Math. These include
trigonometric, logarithmic, exponential, and other functions. For
example, if you want to use the trigonometric function sine, you
would write
Math.sin(1.56)
Note
that all trigonometric methods of Math take arguments in
radians.
The
following table summarizes the Math object's methods.
Table 7.1
Methods of Math
Method
|
Description
|
abs
|
Absolute value
|
sin, cos, tan
|
Standard trigonometric functions;
argument in radians
|
acos, asin, atan, atan2
|
Inverse trigonometric functions; return
values in radians
|
exp, log
|
Exponential and natural logarithm, base
e
|
ceil
|
Returns least integer greater than or
equal to argument
|
floor
|
Returns greatest integer less than or
equal to argument
|
min, max
|
Returns greater or lesser (respectively)
of two arguments
|
pow
|
Exponential; first argument is base,
second is exponent
|
random
|
Returns a random number between 0 and 1.
|
round
|
Rounds argument to nearest integer
|
sqrt
|
Square root
|
Unlike
many other objects, you never create a Math object of your
own. You always use the predefined Math object.
Number Object
The
Number
object has properties for numerical constants, such as maximum
value, not-a-number, and infinity. You cannot change the values of
these properties and you use them as follows:
biggestNum = Number.MAX_VALUE
smallestNum = Number.MIN_VALUE
infiniteNum = Number.POSITIVE_INFINITY
negInfiniteNum = Number.NEGATIVE_INFINITY
notANum = Number.NaN
You
always refer to a property of the predefined Number object as
shown above, and not as a property of a Number object you
create yourself.
The
following table summarizes the Number object's
properties.
Table 7.2
Properties of Number
Property
|
Description
|
MAX_VALUE
|
The largest representable number
|
MIN_VALUE
|
The smallest representable number
|
NaN
|
Special "not a number" value
|
NEGATIVE_INFINITY
|
Special infinite value; returned on
overflow
|
POSITIVE_INFINITY
|
Special negative infinite value;
returned on overflow
|
The
Number prototype provides methods for retrieving information from
Number objects in various formats. The following table summarizes
the methods of Number.prototype.
Table 7.3
Methods of Number.prototype
Method
|
Description
|
toExponential
|
Returns a string representing the
number in exponential notation.
|
toFixed
|
Returns a string representing the
number in fixed-point notation.
|
toPrecision
|
Returns a string representing the
number to a specified precision in fixed-point notation.
|
toSource
|
Returns an object literal
representing the specified Number object; you can use
this value to create a new object. Overrides the
Object.toSource
method.
|
toString
|
Returns a string representing the
specified object. Overrides the
Object.toString
method.
|
valueOf
|
Returns the primitive value of the
specified object. Overrides the
Object.valueOf
method.
|
RegExp Object
The RegExp object
lets you work with regular expressions. It is described in
Chapter 4, "Regular
Expressions."
String Object
The String object is
a wrapper around the string primitive data type. Do not confuse
a string literal with the String object.
For example, the following code creates the string literal
s1 and
also the String object
s2:
s1 = "foo" //creates a string literal
value
s2 = new String("foo") //creates a String object
You can call any of the methods of the
String
object on a string literal value—JavaScript automatically
converts the string literal to a temporary String object,
calls the method, then discards the temporary String object.
You can also use the String.length
property with a string literal.
You should use string literals unless you
specifically need to use a String object,
because String objects
can have counterintuitive behavior. For example:
s1 = "2 + 2" //creates a string literal
value
s2 = new String("2 + 2")//creates a String object
eval(s1) //returns the number 4
eval(s2) //returns the string "2 + 2"
A
String
object has one property, length, that
indicates the number of characters in the string. For example,
the following code assigns x the value 13,
because "Hello, World!" has 13 characters:
myString = "Hello, World!"
x = mystring.length
A
String
object has two types of methods: those that return a variation
on the string itself, such as substring and
toUpperCase, and
those that return an HTML-formatted version of the string, such
as bold and
link.
For example, using the previous example,
both mystring.toUpperCase()
and "hello,
world!".toUpperCase() return the string "HELLO, WORLD!"
The substring method
takes two arguments and returns a subset of the string between
the two arguments. Using the previous example,
mystring.substring(4,
9) returns the string "o, Wo". See the substring
method of the String
object in the
Core JavaScript Reference for more information.
The String object
also has a number of methods for automatic HTML formatting,
such as bold to create
boldface text and link to create a
hyperlink. For example, you could create a hyperlink to a
hypothetical URL with the link method as
follows:
mystring.link("http://www.helloworld.com")
The following table summarizes the methods
of String objects.
Table 7.4
Methods of String Instances
Method
|
Description
|
anchor
|
Creates HTML named anchor.
|
big, blink, bold,
fixed, italics, small,
strike, sub, sup
|
Create HTML formatted string.
|
charAt, charCodeAt
|
Return the character or character
code at the specified position in string.
|
indexOf, lastIndexOf
|
Return the position of specified
substring in the string or last position of specified
substring, respectively.
|
link
|
Creates HTML hyperlink.
|
concat
|
Combines the text of two strings
and returns a new string.
|
fromCharCode
|
Constructs a string from the
specified sequence of Unicode values. This is a method
of the String class, not a String instance.
|
split
|
Splits a String
object into an array of strings by separating the
string into substrings.
|
slice
|
Extracts a section of an string
and returns a new string.
|
substring, substr
|
Return the specified subset of the
string, either by specifying the start and end indexes
or the start index and a length.
|
match, replace, search
|
Work with regular expressions.
|
toLowerCase, toUpperCase
|
Return the string in all lowercase
or all uppercase, respectively.
|